Python Tutorial for Beginners | Part 3
- Ian Vicino
- Jul 21, 2023
- 7 min read
Now, we will finally learn the rest of the data types you will need to make more complex applications. These data types are crucially important if you want to hold multiple numbers and manipulate them. These last five data types can be a bit involved so if you want to learn more about them, please let me know. I would be happy to put up a more involved article about each individual data type but for now I will just give an overview, just enough to get you started having fun with Python. With this knowledge you should be able to attempt more challenging programming applications and that is where Python gets more fun.
Introduction to List, Tuple, Dictionary and Set
The data types we have left to learn are the list, tuple, dictionary, set, and boolean. The list, tuple, dictionary and set are data types that can hold a series of strings, integers, floats, or any combination of the three data types. The boolean will be discussed after we discuss the first four data types. Please see the image below to have a better example of what a list, tuple, dictionary and set look like.
Example 1
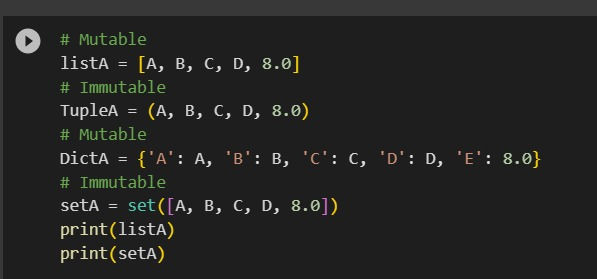
We had previously created some variables containing float values contained in variables A, B, C, and D. I used those variables and put them into a list, tuple, dictionary and set. Some of those data types are mutable, and the others are labeled as immutable.
Mutable vs Immutable data types
A mutable data type is a data type that can be changed. Examples of this are lists, dictionaries, and sets. This means that the contents inside a list, dictionary and set can be added to, removed, or changed without affecting the identity of the data type.
All the other data types, including the tuple are immutable data types. Their contents cannot be changed without effecting the data types identity. This is not so important for what you will actually be coding, but more for how the underlying code works, so I won’t get too far into the difference between immutable and mutable data types. See example below where I use the id function to print out the identity of the variable. If the id is different, then it is a different variable, but if it is the same, then we did not change the identity of the variable.
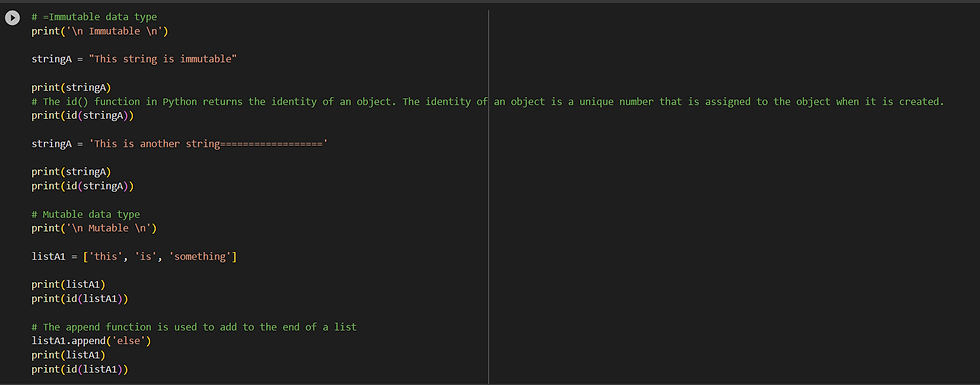
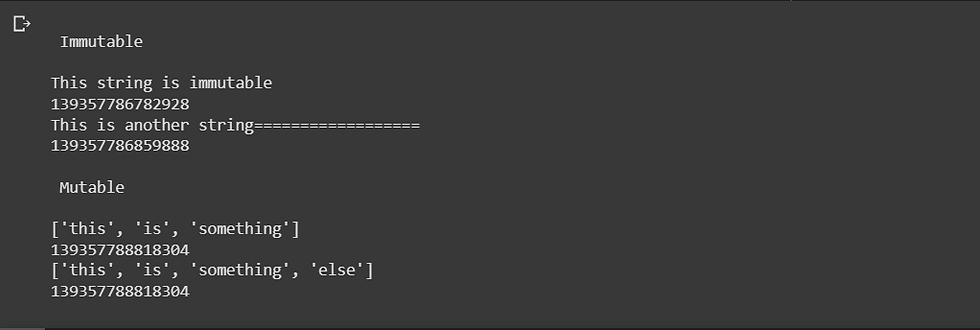
Note: If anything is confusing during this lesson or any other lessons, please do not be afraid to pause and look it up on google. Developers constantly have to use Google or another search engine to remind themselves of something they forgot or learn something new. If you are to advance in programming you should not fear Google or looking something up online. One example of something you may look up is where I used the \n in my string. I won’t tell you what \n does but tell you to look it up. You can paste “python \n” into google and find a good answer as to what it does.
The List
We will first go over my favorite of these four data types, the list. The list is an amazing data type. The list contains any number of integers, floats or string values you put inside it. You can even put a list inside another list, making a list of lists, or nested list. In python a list is initialized by creating a variable and making it equal to a pair of brackets and putting the contents inside the brackets separated by commas. This is shown in the above image where you can see the list named listA1 and that it contains a list of strings. Each entry into a list is separated by a comma, as shown in the example.
After I print the list to the console, I then add to the list using another python function, the .append funciton. The .append function adds what you put in parentheses to the end of the list. If you instead want to add something to the middle of the list, you can denote the location you wish to add by its index. Python starts counting from 0, so if you want to add something after the word ‘is’ for instance, you would use the index value of 2 as shown in the example below.

I inserted into the middle of the list, listA1, by using a different function .insert which requires the index number followed by what you want to insert, in this case the word ‘Inserting…’. There are many other things you can do with lists, but now it is your turn to figure out how to use the list data type. Figure out how to change the word ‘Inserting…’ to the number 64, by searching how to do that on Google, then play around with it. Try to figure out how to delete stuff from lists. At the end of this tutorial I will include a challenge to test your knowledge of lists as well.
The Tuple
The tuple is similar to a list except that it uses parentheses instead of brackets as shown in the below example.
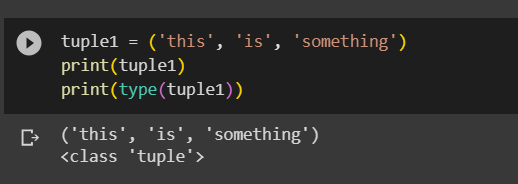
The difference between a tuple and a list is the tuple cannot be changed, it is immutable, as I explained above. If I search “how to append to a tuple” on Google, I come up with this response: “Tuples are immutable, so you cannot append to them. You can, however, create a new tuple that contains the original tuple and the new element.” So in order to change the contents of a tuple, you will need to create a new tuple and add what you want to the new tuple. See example below:
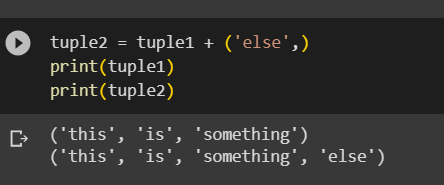
In the example, I added the word ‘else’ by creating a new tuple called tuple2 and adding another tuple to tuple1.
Why would you need a data type that is immutable? Simple, if you want to store something you do not want to be changed, such as a list of passwords, or user names. If you want a list unaltered, create an immutable list.
The Set
I will discuss the dictionary next, but wanted to discuss the set first because it is very similar to a list and tuple except that it does not contain any duplicate entries. If you look at Example 1, shown again below, you can see the list, which contains all the entries that are contained in the set, have two entries for 8.0 but the set outputs only one entry for 8.0. This is because a set combines duplicate entries into one entry.

A set is useful in for the reason it doesn’t allow replicates, as may be needed when storing usernames, or finding the number of unique entries in a list.
The Dictionary
The dictionary is a bit different than the other three data types. The dictionary stores key – value pairs together. It is surprisingly useful in that you can access a value using its key or visa versa. An example of a dictionary can be seen in Example 1, shown above as well, with the variable labeled DictA. In that example, the key is a string value, and the value is the float value we have been using thus far. Play around with this dictionary data type and try and change certain values, or add values to the dictionary. Look up what you want to do online. It is more fun to learn on your own and make mistakes.
The Boolean (Bool)
The boolean, or bool, is a value we have not yet discussed. A bool can either be True or False. It can be returned from a function, or used as a value in a list, tuple, set, or dictionary. We will come across more bool values in future tutorials, most likely when we discuss If statements, so stay tuned for more tutorials. In python the bool values must be capitalized as shown below.

In the code you can see the value of True is colored blue, which is what we are looking for. If you look at the last line of code you can see I then tried to replace the True value with the a False value but did not capitalize the false value. In the code, before I even ran the google colab cell, it was underlined indicating there may be an error with the spelling of the boolean. If you get an error similar to this, the fist thing you should look at is the spelling or in this case the capitalization of what you are trying to do.
We will play around more with each of these data types as we progress through our tutorials, but if you haven’t done so already take some time to play around with each of these data types on your own.
Conclusion
Once you learn about a certain function in Python it can be added to your tool box. Now it is up to your creativity to create something fantastic and fun using what you have learned.
We have learned all the basic data types you need to get started creating with Python, the list, tuple, set, dictionary, boolean as well as previously the integer, float and string values. These will be the data types you will use most during your python journey. Below I will put down a challenge that will test how you might use the list data type, as well as the dictionary data type. If you need a primer to get you learning about these data types, try to solve the challenge below. However, I encourage you to skip this challenge and come up with challenges of your own to test what you know. Make something to show off to your mom, friends, or significant other, make something to save yourself time doing a tedious task, or begin on your dream of creating a program you can sell.
Challenge (generated using OpenAI’s chatGPT)
Challenge Name: "Holiday Planner"
_______________________________________________________________________
Description: You are planning a holiday trip, and you need to organize various aspects of your journey. Your task is to create a Python program that allows users to add, view, and modify their travel plans using lists and dictionaries.
Requirements:
The program should provide a menu with the following options:
a. Add a new destination
b. View all destinations
c. Modify a destination
d. Exit the program
Each destination should have the following attributes:
Name of the destination
Date of departure
Duration of the trip (in days)
List of items to pack
Users should be able to perform the following actions:
a. Add a new destination:
Prompt the user to enter the destination name, date of departure, duration, and items to pack.
Add this information as a new entry in the list of destinations.
b. View all destinations:
Display a formatted list of all destinations with their respective details.
c. Modify a destination:
Prompt the user to enter the name of the destination they want to modify.
Allow the user to update any of the attributes of that destination (date, duration, or items to pack).
d. Exit the program:
End the program execution.
Hints:
Use a list to store multiple destination dictionaries.
Each dictionary will represent a single destination with its attributes as key-value pairs.
You can use a loop and conditional statements to implement the menu and perform the corresponding actions based on the user's choice.
Comments