Python Tutorial for Beginners | Part 2
- Ian Vicino
- Jul 11, 2023
- 5 min read
Updated: Sep 19, 2023
Building off of part one of this tutorial series, we will discuss the error messages you may have experienced in part 1 of this tutorial, and you will experience in the future, as well as different data types while continuing to code.
Error Messages
To begin, please open up the google colab document you created in Part 1 as we will be working using the same work book. That is one advantage of using google colab, the fact that each block of code can be run individually so we do not have to open up new documents with each lesson.

In the code you can see in the image above, you can see multiple changes to the code we ran during part 1. The first notable change is the hashtag (#) followed by the words “Part 1” and “Part 2”. These are called comments and are skipped when you run the block of code. These comments are how developers explain what a line of code does so others can understand the code better. You can write whatever you feel is needed after the # mark. Another way to comment out code is by using three sets of quotation marks (ex: “““Part 1”””) which is beneficial if you want to comment out multiple lines of text as can be seen below.
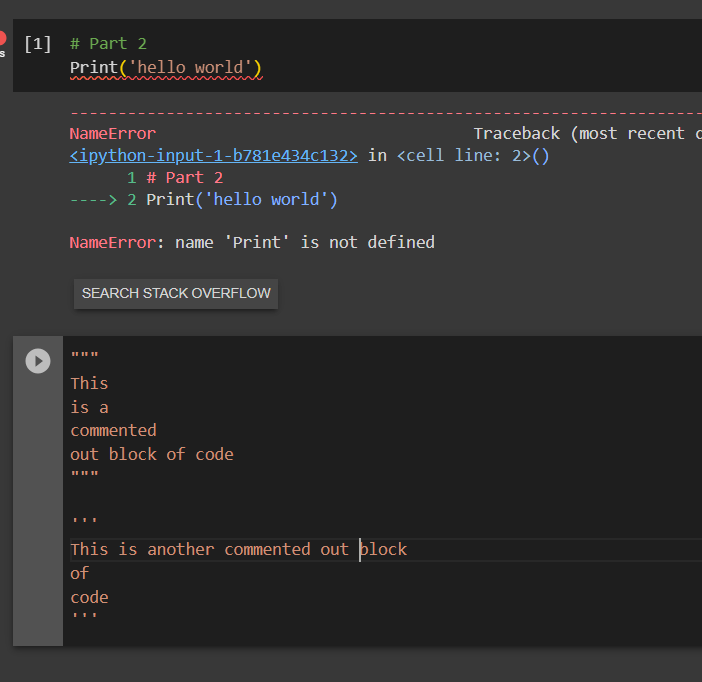
The other notable change you may have seen is the error comment seen in both images. This error shows what line of code caused the error as well as what the source of the error may have been. These error messages, in this case a NameError, are fantastic ways to troubleshoot your code. They will not directly show you how to fix your code, but can help you figure out what to look at to fix your code. In this case we are pointed to look at the line that says Print(‘hello world’). It says the name 'Print' is not definedwhich leads us to look at the word “print”. In this case you should notice the P in the word Print is capitalized which is not correct. If you lowercase the P you should be able to resolve the error.
I realize this was a simple case study that could be identified and resolved quickly without even looking at the error message, but these error messages will become really important as you continue to code. As you advance, you will notice errors that do not show up with an error code, instead your program outputs something you did not expect, and wish your program had shown an error code. They will become very important to you as you troubleshoot your programming. I will go further into troubleshooting if you need some help with it in the future, just let me know, but the first step of troubleshooting should always involve looking at these error codes.
Data Types: Strings, Integers and Floats
Now let us move on to learn more about data types. Previously you learned about strings. This is the first data type you will learn about. Strings, if you remember correctly, are a sequence of characters surrounded by quotation marks, either single or double quotation marks, as you used when you wrote “Hello World!”. These are generally used when you want to output a line of text.
The next data type we will look at are integers and floats. Integers are any number without a decimal point. Examples are the numbers 1, 256, 2837483165441035465, and 4. As soon as you add a decimal point to a number it becomes a floating-point numberor float. So, the number 4 is an integer but the number 4. or the number 2837483165.441035465 arefloats. If you add, multiply, subtract or divide an integer to a float the calculation will output a float. This can be seen in the code below. As an additional point, if you divide any number by any other number the output will be a float, unless you specify it to become an integer, I will show more about this in the next lesson.
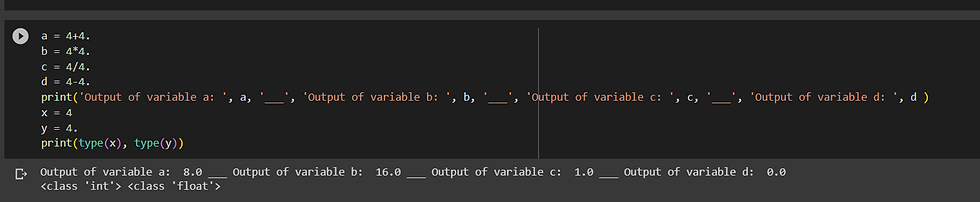
The code also shows some new concepts including variables and a method to output the data type of a variable. In the code you can see the variables A, B, C, and D. Variables are like boxes where you store data, in this case integer and float numbers. This is where Python gets really cool, and easy to use. Most programming languages require a developer to define what data type will enter into the variable box, but Python is a dynamic typed language meaning it figures out what data type is in the variable in the background. I will get into more on that in future lessons, but you can learn more about how Python works by playing around with these variables. Wait, before you begin playing around with Python first I have one more thing to teach you.
Get User Input
I need to teach you how to get user input. Not only can you program in the contents of a variable as we did above, but you can get a user to input data into a variable. The way we get user input is by using the built-in input class. You can use the input class by typing input followed by parentheses as shown in code below.
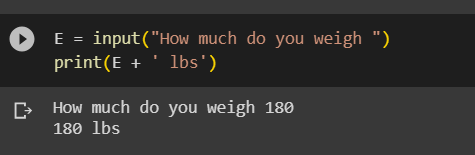
In the code above you can the variable E will contain the text input by the user after being prompted with the question “How much do you weigh.” The contents of E will be a string, if you do not believe me try it out yourself, which allows us to add ‘ lbs’ to the end of the string contained in the variable E. Python allows us to add strings together and actually multiply a string by an integer. (Try that out for yourself) This is what I did in the last line of text which produced “180 lbs” as the output. Now you have all the tools you need to solve the challenge below. The challenge will involve a bit of math, but luckily for you the computer you are reading this through is a great calculator. You just need to figure out how to use it to get the result you want.
If you need any help, or clarification during my lesson in order to solve this challenge, please let me know! Any feedback is appreciated. In the next lesson we will be going over the data types of lists, tuples, dictionaries, sets and bools completing our knowledge of all the basic data types in Python. Then we can tackle some loops, which will just increase the fun. Whenever you are bored, pick up your computer and code, it will benefit you in the long run.
Coding Challenge (generated using OpenAI’s chatGPT)
I have a programming challenge so you can learn more about variables, and data types we have just gone over. I am not going to walk you through this challenge, instead I want you to take it on yourself and have fun.
Challenge: Calculate Body Mass Index (BMI) - Write a Python program that calculates the Body Mass Index (BMI) based on the user's weight and height.
The BMI is calculated using the formula: BMI = weight / (height * height)
Instructions:
Prompt the user to enter their weight in kilograms (as a float value).
Prompt the user to enter their height in meters (as a float value).
Calculate the BMI using the formula above and assign it to a variable.
Print the calculated BMI.
Comments